本文介紹Java運行時實現函數實例生命周期回調的方法。
背景信息
當您實現并配置函數實例生命周期回調后,函數計算系統將在相關實例生命周期事件發生時調用對應的回調程序。函數實例生命周期涉及Initializer、PreStop和PreFreeze三種回調。Java運行時已支持三種回調方式。更多信息,請參見函數實例生命周期回調。
函數實例生命周期回調程序與正常調用請求計費規則一致,但其執行日志只能在函數日志、實例日志或高級日志中查詢,調用請求列表不會展示回調程序日志。具體操作,請參見查看實例生命周期回調函數日志。
回調方法簽名
Initializer回調簽名
初始化回調程序(Initializer回調)是在函數實例啟動成功之后,運行請求處理程序(Handler)之前執行。函數計算保證在一個實例生命周期內,成功且只成功執行一次Initializer回調。例如您的Initializer回調第一次執行失敗了,系統會重試,直到成功為止,然后再執行您的請求處理程序。因此,您在實現Initializer回調時,需要保證它被重復調用時的正確性。
Initializer回調只有一個context輸入參數,使用方法同事件請求處理程序。
initialize
方法,接口定義如下。package com.aliyun.fc.runtime;
import java.io.IOException;
/**
* This is the interface for the initialization operation
*/
public interface FunctionInitializer {
/**
* The interface to handle a function compute initialize request
*
* @param context The function compute initialize environment context object.
* @throws IOException IOException during I/O handling
*/
void initialize(Context context) throws IOException;
}
PreStop回調簽名
preStop
方法,接口定義如下。package com.aliyun.fc.runtime;
import java.io.IOException;
/**
* This is the interface for the preStop operation
*/
public interface PreStopHandler {
/**
* The interface to handle a function compute preStop request
*
* @param context The function compute preStop environment context object.
* @throws IOException IOException during I/O handling
*/
void preStop(Context context) throws IOException;
}
PreFreeze回調簽名
preFreeze
方法,接口定義如下。package com.aliyun.fc.runtime;
import java.io.IOException;
/**
* This is the interface for the preFreeze operation
*/
public interface PreFreezeHandler {
/**
* The interface to handle a function compute preFreeze request
*
* @param context The function compute preFreeze environment context object.
* @throws IOException IOException during I/O handling
*/
void preFreeze(Context context) throws IOException;
}
簡單示例:流式事件請求處理程序
package example;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import com.aliyun.fc.runtime.Context;
import com.aliyun.fc.runtime.StreamRequestHandler;
import com.aliyun.fc.runtime.FunctionInitializer;
import com.aliyun.fc.runtime.PreFreezeHandler;
import com.aliyun.fc.runtime.PreStopHandler;
public class App implements StreamRequestHandler, FunctionInitializer, PreFreezeHandler, PreStopHandler {
@Override
public void initialize(Context context) throws IOException {
context.getLogger().info("initialize start ...");
}
@Override
public void handleRequest(
InputStream inputStream, OutputStream outputStream, Context context) throws IOException {
context.getLogger().info("handlerRequest ...");
outputStream.write(new String("hello world\n").getBytes());
}
@Override
public void preFreeze(Context context) throws IOException {
context.getLogger().info("preFreeze start ...");
}
@Override
public void preStop(Context context) throws IOException {
context.getLogger().info("preStop start ...");
}
}
配置生命周期回調函數
通過控制臺配置
[包名].[類名]::[方法名]
。示例如下: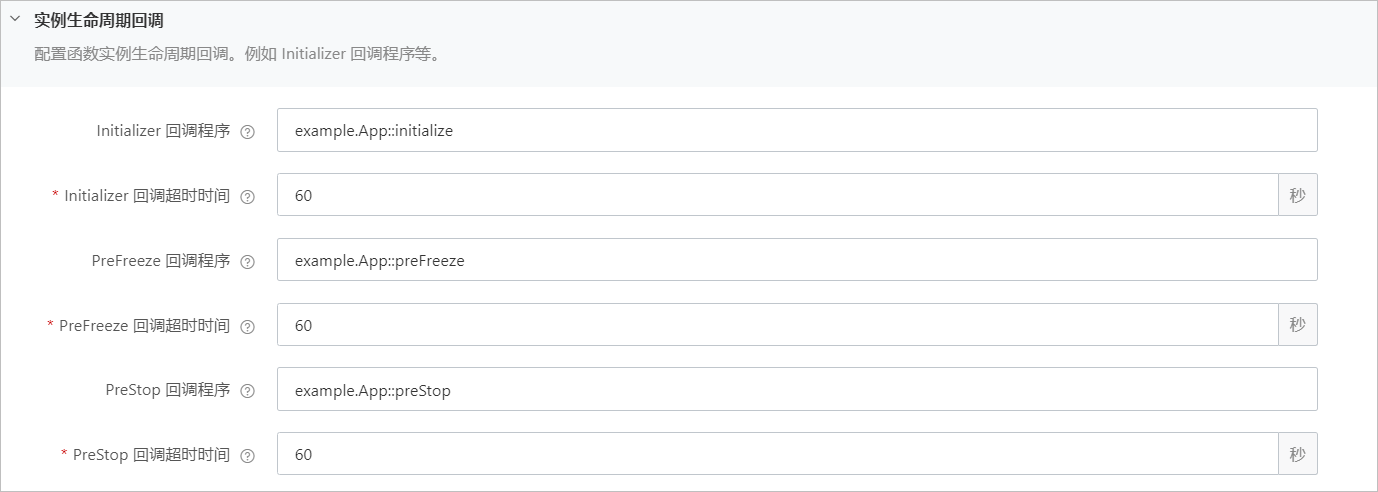
- Initializer回調程序:設置為
example.App::initialize
,表示實現example
包中App.java文件里的initialize
方法。 - PreFreeze回調程序:設置為
example.App::preFreeze
,表示實現example
包中App.java文件里的preFreeze
方法。 - PreStop回調程序:設置為
example.App::preStop
,表示實現example
包中App.java文件里的preStop
方法。
通過Serverless Devs配置
s.yaml
配置文件中添加Initializer 回調程序、PreFreeze 回調程序和PreStop 回調程序。
- Initializer回調配置
在function配置下添加initializer和initializationTimeout兩個字段。
- PreFreeze回調配置
在function配置下添加instanceLifecycleConfig.preFreeze字段,包括handler和timeout兩個字段。
- PreStop回調配置
在function配置下添加instanceLifecycleConfig.preStop字段,包括handler和timeout兩個字段。
具體的示例如下所示。
edition: 1.0.0
name: hello-world # 項目名稱
access: default # 密鑰別名
vars: # 全局變量
region: cn-shanghai # 地域
service:
name: fc-example
description: 'fc example by serverless devs'
services:
helloworld: # 業務名稱/模塊名稱
component: fc
actions: # 自定義執行邏輯
pre-deploy: # 在deploy之前運行
- run: mvn package # 要運行的命令行
path: ./ # 命令行運行的路徑
props: # 組件的屬性值
region: ${vars.region}
service: ${vars.service}
function:
name: java8-lifecycle-hook-demo
description: 'fc example by serverless devs'
runtime: java8
codeUri: ./target
handler: example.App::handleRequest
memorySize: 128
timeout: 60
initializationTimeout: 60
initializer: example.App::initialize
instanceLifecycleConfig:
preFreeze:
handler: example.App::preFreeze
timeout: 30
preStop:
handler: example.App::preStop
timeout: 30
關于Serverless Devs的YAML配置規范,請參見Serverless Devs操作命令。
查看實例生命周期回調函數日志
您可以通過函數日志功能查看回調函數日志。
示例程序
- java11-mysql:函數計算提供的Initializer回調和PreStop回調的示例程序。
該示例為您展示了如何使用Java運行時的Initializer回調從環境變量中獲取數據庫配置并創建MySQL連接,以及如何使用PreStop回調負責關閉MySQL連接。